https://www.acmicpc.net/problem/1303
1303번: 전쟁 - 전투
첫째 줄에는 전쟁터의 가로 크기 N, 세로 크기 M(1 ≤ N, M ≤ 100)이 주어진다. 그 다음 두 번째 줄에서 M+1번째 줄에는 각각 (X, Y)에 있는 병사들의 옷색이 띄어쓰기 없이 주어진다. 모든 자리에는
www.acmicpc.net
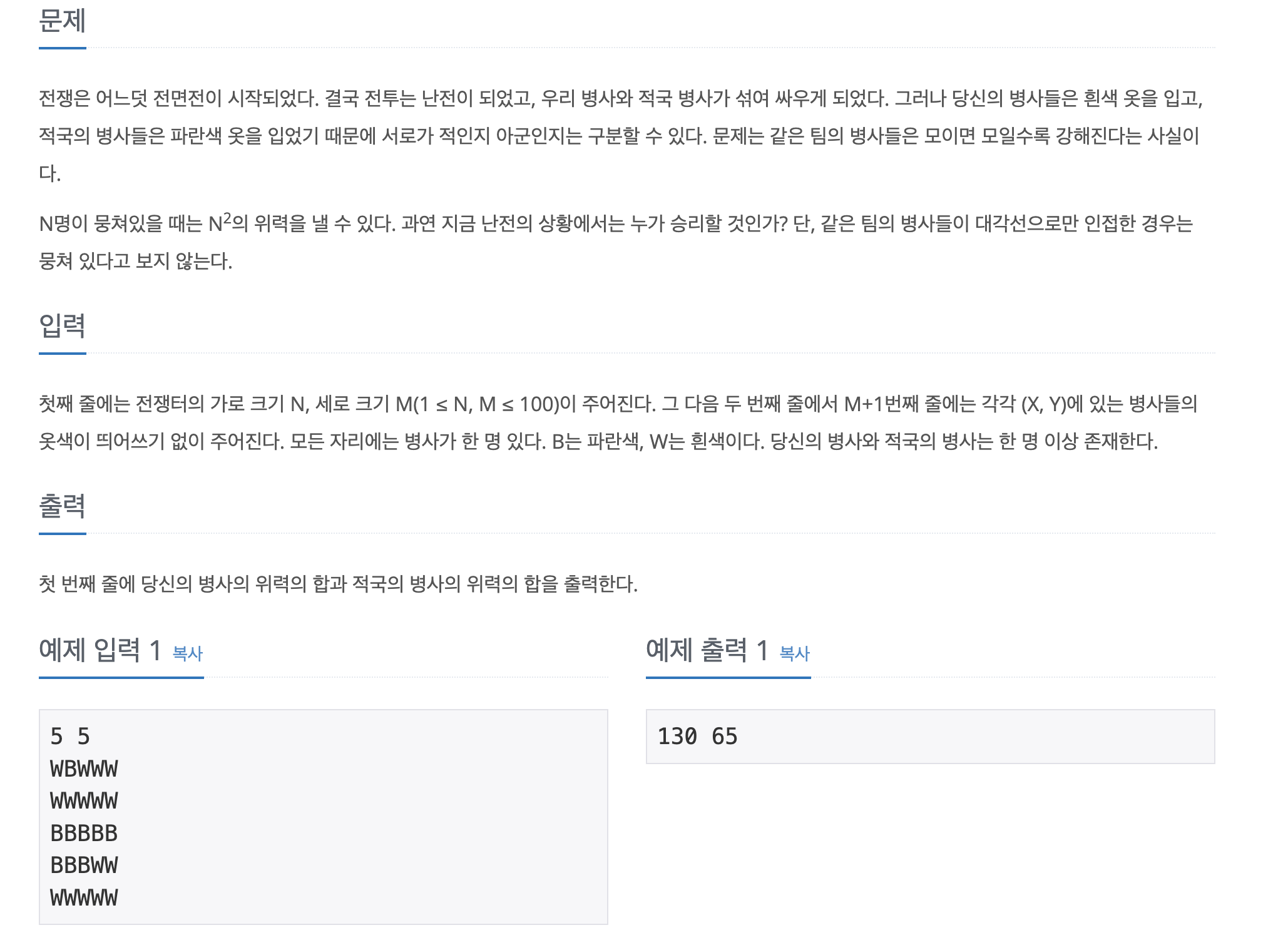
풀이 >
그래프 탐색에서 큐를 사용해서 BFS 로 해결 가능하다.
W, B 각각 병사에 대해 탐색하고 방문 표시를 해주면 된다.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.Queue;
public class Main {
static int[] dirI = {1, -1, 0, 0};
static int[] dirJ = {0, 0, 1, -1};
static boolean[][] visited;
static String[][] matrix;
static int N;
static int M;
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String[] tmp = reader.readLine().split(" ");
M = Integer.parseInt(tmp[0]);
N = Integer.parseInt(tmp[1]);
matrix = new String[N][M];
visited = new boolean[N][M];
for(int i = 0; i<N; i++) {
matrix[i] = reader.readLine().split("");
}
int B = 0;
int W = 0;
Queue<int[]> queueB = new LinkedList<>();
Queue<int[]> queueW = new LinkedList<>();
for(int i = 0; i<N; i++) {
for(int j = 0; j<M; j++) {
if (!visited[i][j]) {
visited[i][j] = true;
if ("B".equals(matrix[i][j])) {
queueB.add(new int[]{i, j});
} else {
queueW.add(new int[]{i, j});
}
}
B += checkArmy(queueB, "B");
W += checkArmy(queueW, "W");
}
}
System.out.println(W +" " + B);
}
static public int checkArmy(Queue<int[]> queue, String team) {
int count = 0;
while(!queue.isEmpty()) {
int[] next = queue.poll();
int qi = next[0]; int qj = next[1];
count++;
for(int k = 0; k<4; k++) {
int nexti = dirI[k] + qi;
int nextj = dirJ[k] + qj;
// 상하좌우 병사 확인
if (nexti >= 0 && nexti < N && nextj >= 0 && nextj < M && !visited[nexti][nextj] && matrix[nexti][nextj].equals(team)) {
visited[nexti][nextj] = true;
queue.add(new int[]{nexti, nextj});
}
}
}
return count*count;
}
}
'알고리즘 > 백준' 카테고리의 다른 글
[백준] 9471번 피사노 주기 (0) | 2023.06.07 |
---|---|
[백준] 1655번. 가운데를 말해요 (Java) (0) | 2023.06.07 |
[백준] 1629번 곱셈 (0) | 2023.02.08 |
[백준] 1309번 : 동물원 (0) | 2023.01.19 |
[백준] 1052. 물병 (0) | 2023.01.12 |